There comes a time in every Docker container‘s lifecycle when it crashes unexpectedly. As the container owner, you’ll find yourself wishing you had a backup snapshot, like a magic time machine that could take you back two minutes to when your container was up, running, and happy.
This exact scenario happened to me recently. The website you’re currently reading runs in a Docker container on an Ubuntu Linux server. And yes, you guessed it—I didn’t have any container snapshot ready when disaster struck.
Determined not to let this happen again, I decided to consult ChatGPT for a solution. After a few productive exchanges, I crafted a script to back up my Docker containers efficiently. Here, I’ll share the script and explain how to use it to safeguard your containers.
Why Back Up Your Docker Container?
Backing up Docker containers ensures you can restore them in case of a crash, accidental deletion, or corruption. While Docker itself doesn’t offer a built-in backup mechanism, with the right tools and approach, you can create and manage backups effortlessly. By setting up regular backups, you’re taking a proactive step to secure your containerized applications and data.
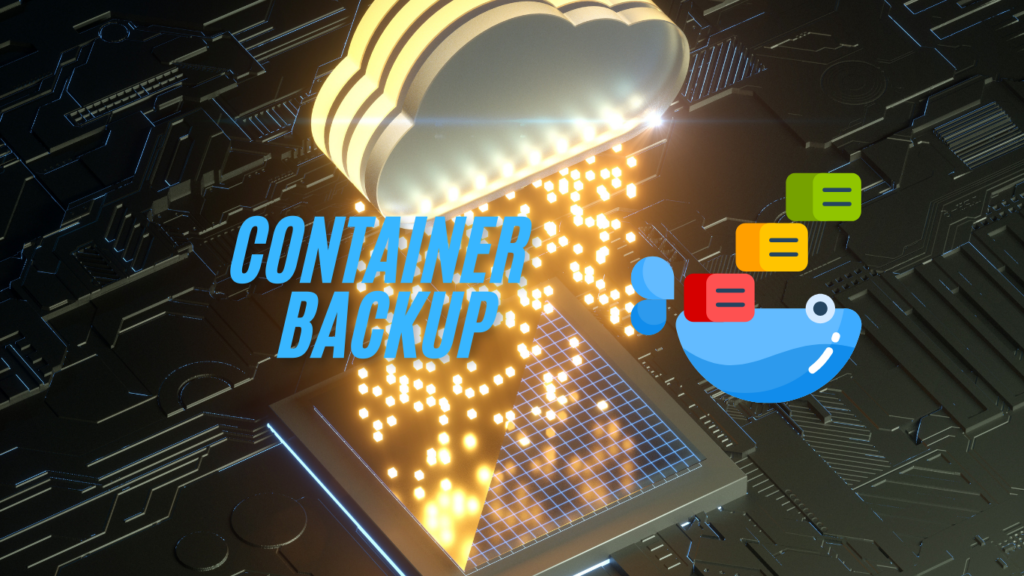
A Simple Docker Backup Script
Below is the backup script I developed. This script allows you to back up a container based on its name, image name, or container ID. It also includes a feature to retain only the last three backups in a folder, ensuring that storage space isn’t overwhelmed.
#!/bin/bash
# Variables
BACKUP_DIR="/path/to/backup/folder" # Replace with your backup directory
CONTAINER_NAME="$1" # Container name, image name, or ID
DATE=$(date +"%Y%m%d%H%M%S")
BACKUP_FILE="$BACKUP_DIR/${CONTAINER_NAME}_backup_$DATE.tar.gz"
# Create the backup directory if it doesn't exist
mkdir -p "$BACKUP_DIR"
# Check if container exists
docker ps -a --format "{{.Names}}" | grep -q "$CONTAINER_NAME"
if [ $? -ne 0 ]; then
echo "Error: Container '$CONTAINER_NAME' not found."
exit 1
fi
# Create the backup
echo "Creating backup for container '$CONTAINER_NAME'..."
docker export "$CONTAINER_NAME" | gzip > "$BACKUP_FILE"
echo "Backup saved to $BACKUP_FILE"
# Retain only the last 3 backups
BACKUP_COUNT=$(ls "$BACKUP_DIR" | grep "$CONTAINER_NAME" | wc -l)
if [ "$BACKUP_COUNT" -gt 3 ]; then
echo "Cleaning up old backups..."
ls -t "$BACKUP_DIR" | grep "$CONTAINER_NAME" | tail -n +4 | xargs -I {} rm "$BACKUP_DIR/{}"
echo "Old backups cleaned."
fi
How to Use the Script
1. Save the Script: Copy the script into a file, e.g., backup_docker.sh
, and make it executable using:
chmod +x backup_docker.sh
2. Run the Script: Use the script by specifying the container name, image name, or container ID as an argument:
./backup_docker.sh my_container
3. Automate with Cron: To automate backups, add the script to your cron jobs:
crontab -e
Add the following line to back up every day at 2 AM:
0 2 * * * /path/to/backup_docker.sh my_container
Why Keep Only the Last 3 Backups?
Storage can quickly fill up if backups accumulate unchecked. By retaining only the three most recent backups, this script ensures efficient storage management without sacrificing safety.
Try It Yourself!
If you’re curious to see this backup method in action, why not try it yourself? Start by creating a new droplet on DigitalOcean. Set up a dummy Docker container and use this backup script to create snapshots. It’s a safe and practical way to get hands-on experience with Docker backups. Seeing the process firsthand will give you confidence in implementing this method for your production containers.
Regular backups are an essential practice for anyone running Docker containers. This simple script offers a reliable way to create and manage backups, giving you peace of mind and a quick recovery option if things go awry.
Set up your Docker container backup script today and ensure your Docker containers are always ready to bounce back from unexpected issues!